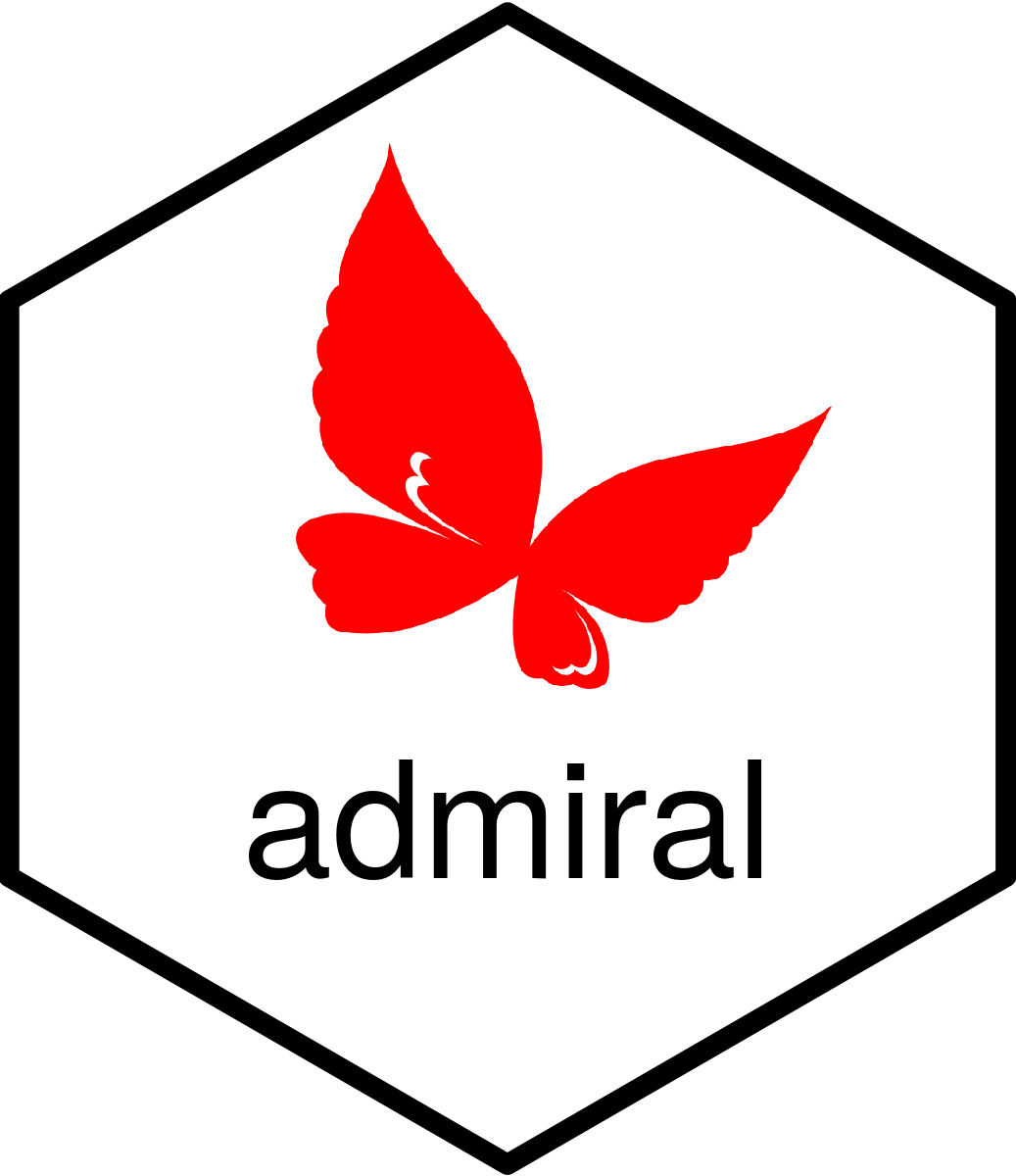
Add a parameter for lab differentials converted to absolute values
Source:R/derive_param_wbc_abs.R
derive_param_wbc_abs.Rd
Add a parameter by converting lab differentials from fraction or percentage to absolute values
Usage
derive_param_wbc_abs(
dataset,
by_vars,
set_values_to,
get_unit_expr,
wbc_unit = "10^9/L",
wbc_code = "WBC",
diff_code,
diff_type = "fraction"
)
Arguments
- dataset
-
Input dataset
The variables specified by the
by_vars
argument are expected to be in the dataset.PARAMCD
, andAVAL
are expected as well.The variable specified by
by_vars
andPARAMCD
must be a unique key of the input dataset, and to the parameters specified bywbc_code
anddiff_code
. - by_vars
-
Grouping variables
Permitted Values: list of variables created by
exprs()
e.g.exprs(USUBJID, VISIT)
- set_values_to
-
Variables to set
A named list returned by
exprs()
defining the variables to be set for the new parameter, e.g.exprs(PARAMCD = "LYMPH", PARAM = "Lymphocytes Abs (10^9/L)")
is expected. - get_unit_expr
-
An expression providing the unit of the parameter
The result is used to check the units of the input parameters.
Permitted Values: a variable containing unit from the input dataset, or a function call, for example,
get_unit_expr = extract_unit(PARAM)
. - wbc_unit
-
A string containing the required unit of the WBC parameter
Default:
"10^9/L"
- wbc_code
-
White Blood Cell (WBC) parameter
The observations where
PARAMCD
equals the specified value are considered as the WBC absolute results to use for converting the differentials.Default:
"WBC"
Permitted Values: character value
- diff_code
-
white blood differential parameter
The observations where
PARAMCD
equals the specified value are considered as the white blood differential lab results in fraction or percentage value to be converted into absolute value. - diff_type
-
A string specifying the type of differential
Permitted Values:
"percent"
,"fraction"
Default:fraction
Details
If diff_type
is "percent"
, the analysis value of the new parameter is derived as
$$\frac{White Blood Cell Count * Percentage Value}{100}$$
If diff_type
is "fraction"
, the analysis value of the new parameter is derived as
$$White Blood Cell Count * Fraction Value$$
New records are created for each group of records (grouped by by_vars
) if 1) the white blood
cell component in absolute value is not already available from the input dataset, and 2) the
white blood cell absolute value (identified by wbc_code
) and the white blood cell differential
(identified by diff_code
) are both present.
See also
BDS-Findings Functions for adding Parameters/Records:
default_qtc_paramcd()
,
derive_expected_records()
,
derive_extreme_event()
,
derive_extreme_records()
,
derive_locf_records()
,
derive_param_bmi()
,
derive_param_bsa()
,
derive_param_computed()
,
derive_param_doseint()
,
derive_param_exist_flag()
,
derive_param_exposure()
,
derive_param_framingham()
,
derive_param_map()
,
derive_param_qtc()
,
derive_param_rr()
,
derive_summary_records()
Examples
library(tibble)
test_lb <- tribble(
~USUBJID, ~PARAMCD, ~AVAL, ~PARAM, ~VISIT,
"P01", "WBC", 33, "Leukocyte Count (10^9/L)", "CYCLE 1 DAY 1",
"P01", "WBC", 38, "Leukocyte Count (10^9/L)", "CYCLE 2 DAY 1",
"P01", "LYMLE", 0.90, "Lymphocytes (fraction of 1)", "CYCLE 1 DAY 1",
"P01", "LYMLE", 0.70, "Lymphocytes (fraction of 1)", "CYCLE 2 DAY 1",
"P01", "ALB", 36, "Albumin (g/dL)", "CYCLE 2 DAY 1",
"P02", "WBC", 33, "Leukocyte Count (10^9/L)", "CYCLE 1 DAY 1",
"P02", "LYMPH", 29, "Lymphocytes Abs (10^9/L)", "CYCLE 1 DAY 1",
"P02", "LYMLE", 0.87, "Lymphocytes (fraction of 1)", "CYCLE 1 DAY 1",
"P03", "LYMLE", 0.89, "Lymphocytes (fraction of 1)", "CYCLE 1 DAY 1"
)
derive_param_wbc_abs(
dataset = test_lb,
by_vars = exprs(USUBJID, VISIT),
set_values_to = exprs(
PARAMCD = "LYMPH",
PARAM = "Lymphocytes Abs (10^9/L)",
DTYPE = "CALCULATION"
),
get_unit_expr = extract_unit(PARAM),
wbc_code = "WBC",
diff_code = "LYMLE",
diff_type = "fraction"
)
#> # A tibble: 11 × 6
#> USUBJID PARAMCD AVAL PARAM VISIT DTYPE
#> <chr> <chr> <dbl> <chr> <chr> <chr>
#> 1 P01 WBC 33 Leukocyte Count (10^9/L) CYCLE 1 DAY 1 NA
#> 2 P01 WBC 38 Leukocyte Count (10^9/L) CYCLE 2 DAY 1 NA
#> 3 P01 LYMLE 0.9 Lymphocytes (fraction of 1) CYCLE 1 DAY 1 NA
#> 4 P01 LYMLE 0.7 Lymphocytes (fraction of 1) CYCLE 2 DAY 1 NA
#> 5 P01 ALB 36 Albumin (g/dL) CYCLE 2 DAY 1 NA
#> 6 P02 WBC 33 Leukocyte Count (10^9/L) CYCLE 1 DAY 1 NA
#> 7 P02 LYMPH 29 Lymphocytes Abs (10^9/L) CYCLE 1 DAY 1 NA
#> 8 P02 LYMLE 0.87 Lymphocytes (fraction of 1) CYCLE 1 DAY 1 NA
#> 9 P03 LYMLE 0.89 Lymphocytes (fraction of 1) CYCLE 1 DAY 1 NA
#> 10 P01 LYMPH 29.7 Lymphocytes Abs (10^9/L) CYCLE 1 DAY 1 CALCULATION
#> 11 P01 LYMPH 26.6 Lymphocytes Abs (10^9/L) CYCLE 2 DAY 1 CALCULATION