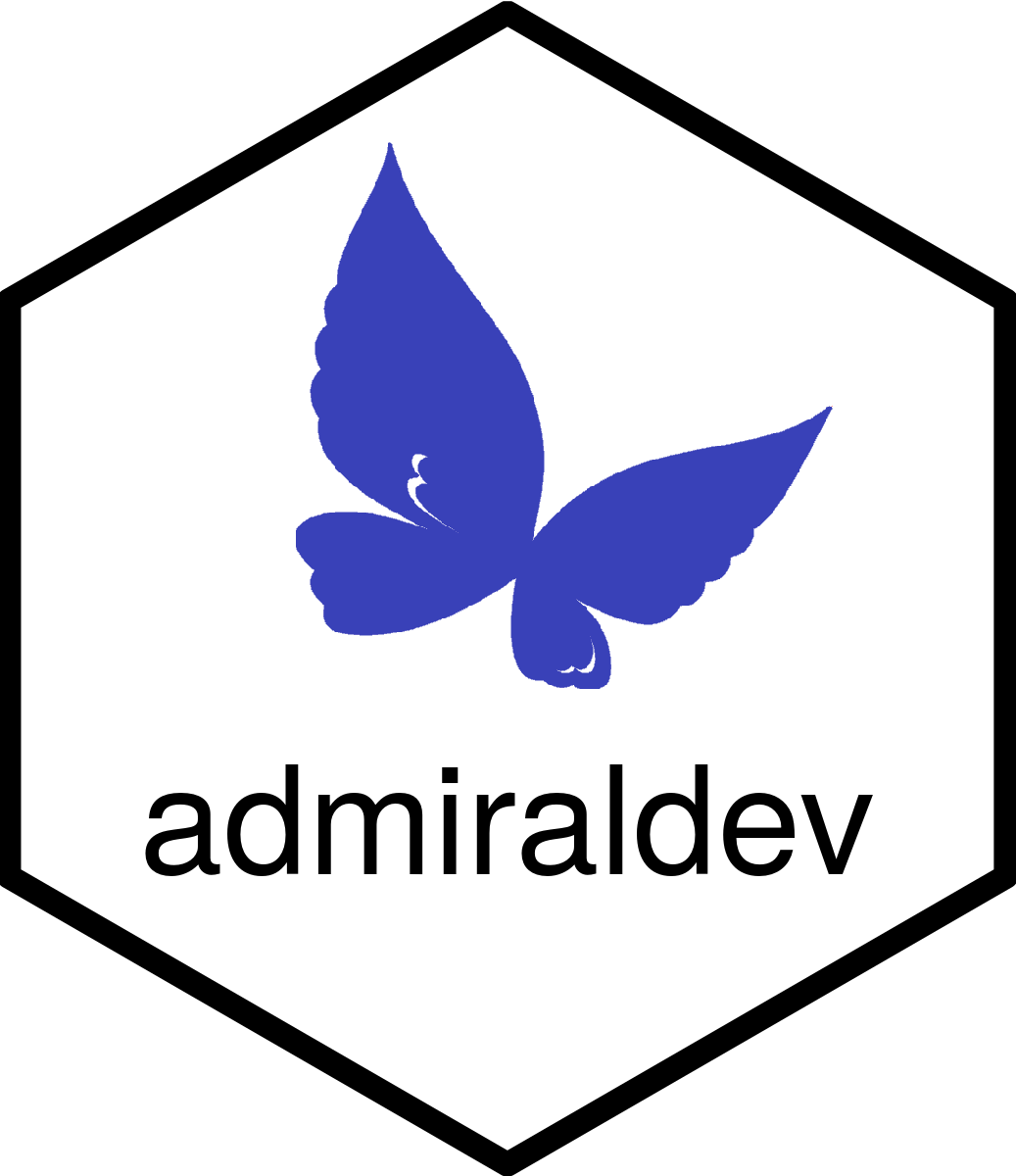
Is an Argument a List of Objects of a Specific S3 Class or Type?
Source:R/assertions.R
assert_list_of.Rd
Checks if an argument is a list
of objects inheriting from the S3 class or type specified.
Usage
assert_list_of(
arg,
cls,
named = FALSE,
optional = TRUE,
arg_name = rlang::caller_arg(arg),
message = NULL,
class = "assert_list_of",
call = parent.frame()
)
Arguments
- arg
A function argument to be checked
- cls
The S3 class or type to check for
- named
If set to
TRUE
, an error is issued if not all elements of the list are named.- optional
Is the checked argument optional? If set to
FALSE
andarg
isNULL
then an error is thrown- arg_name
string indicating the label/symbol of the object being checked.
- message
string passed to
cli::cli_abort(message)
. WhenNULL
, default messaging is used (see examples for default messages)."{arg_name}"
can be used in messaging.- class
Subclass of the condition.
- call
The execution environment of a currently running function, e.g.
call = caller_env()
. The corresponding function call is retrieved and mentioned in error messages as the source of the error.You only need to supply
call
when throwing a condition from a helper function which wouldn't be relevant to mention in the message.Can also be
NULL
or a defused function call to respectively not display any call or hard-code a code to display.For more information about error calls, see Including function calls in error messages.
Value
The function throws an error if arg
is not a list or if arg
is a list but
its elements are not objects inheriting from class
or of type class
.
Otherwise, the input is returned invisibly.
See also
Checks for valid input and returns warning or errors messages:
assert_atomic_vector()
,
assert_character_scalar()
,
assert_character_vector()
,
assert_data_frame()
,
assert_date_vector()
,
assert_expr()
,
assert_expr_list()
,
assert_filter_cond()
,
assert_function()
,
assert_integer_scalar()
,
assert_list_element()
,
assert_logical_scalar()
,
assert_named()
,
assert_numeric_vector()
,
assert_one_to_one()
,
assert_param_does_not_exist()
,
assert_s3_class()
,
assert_same_type()
,
assert_symbol()
,
assert_unit()
,
assert_vars()
,
assert_varval_list()
Examples
example_fun <- function(list) {
assert_list_of(list, "data.frame")
}
example_fun(list(mtcars, iris))
try(example_fun(list(letters, 1:10)))
#> Error in example_fun(list(letters, 1:10)) :
#> Each element of the list in argument `list` must be class/type
#> <data.frame>.
#> ℹ But, element 1 is a character vector, and element 2 is an integer vector
try(example_fun(c(TRUE, FALSE)))
#> Error in example_fun(c(TRUE, FALSE)) :
#> Argument `list` must be class <list>, but is a logical vector.
example_fun2 <- function(list) {
assert_list_of(list, "numeric", named = TRUE)
}
try(example_fun2(list(1, 2, 3, d = 4)))
#> Error in example_fun2(list(1, 2, 3, d = 4)) :
#> All elements of `list` argument must be named.
#> ℹ The indices of the unnamed elements are 1, 2, and 3