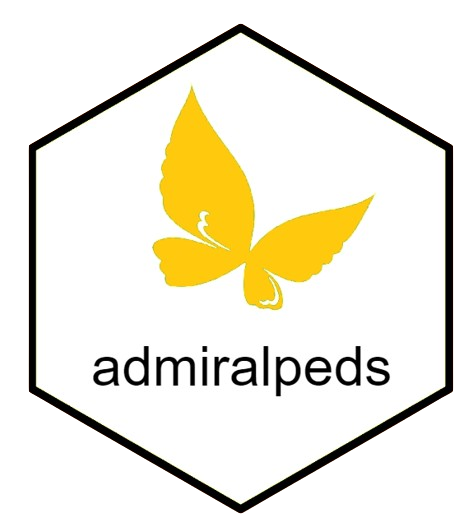
Derive Anthropometric indicators (Z-Scores/Percentiles-for-Height/Length) based on Standard Growth Charts
Source:R/derive_params_growth_height.R
derive_params_growth_height.Rd
Derive Anthropometric indicators (Z-Scores/Percentiles-for-Height/Length) based on Standard Growth Charts for Weight by Height/Length
Usage
derive_params_growth_height(
dataset,
sex,
height,
height_unit,
meta_criteria,
parameter,
analysis_var,
who_correction = FALSE,
set_values_to_sds = NULL,
set_values_to_pctl = NULL
)
Arguments
- dataset
Input dataset
The variables specified in
sex
,height
,height_unit
,parameter
,analysis_var
are expected to be in the dataset.- sex
Sex
A character vector is expected.
Expected Values:
M
,F
- height
Current Height/length
A numeric vector is expected. Note that this is the actual height/length at the current visit.
- height_unit
Height/Length Unit A character vector is expected.
Expected values:
cm
- meta_criteria
Metadata dataset
A metadata dataset with the following expected variables:
HEIGHT_LENGTH
,HEIGHT_LENGTHU
,SEX
,L
,M
,S
The dataset can be derived from WHO or user-defined datasets. The WHO growth chart metadata datasets are available in the package and will require small modifications.
Datasets
who_wt_for_lgth_boys
/who_wt_for_lgth_girls
are applicable for subject age < 730.5 days.If the
height
value fromdataset
falls between twoHEIGHT_LENGTH
values inmeta_criteria
, then theL
/M
/S
values that are chosen/mapped will be theHEIGHT_LENGTH
that has the smaller absolute difference to the value inheight
. e.g. If dataset has a current age of 50.49 cm, and the metadata contains records for 50 and 51 cm, theL
/M
/S
corresponding to the 50 cm record will be used.HEIGHT_LENGTH
- Height/LengthHEIGHT_LENGTHU
- Height/Length UnitSEX
- SexL
- Power in the Box-Cox transformation to normalityM
- MedianS
- Coefficient of variation
- parameter
Anthropometric measurement parameter to calculate z-score or percentile
A condition is expected with the input dataset
VSTESTCD
/PARAMCD
for which we want growth derivations:e.g.
parameter = VSTESTCD == "WEIGHT"
.There is WHO metadata available for Weight available in the
admiralpeds
package. Weight measures are expected to be in the unit "kg".- analysis_var
Variable containing anthropometric measurement
A numeric vector is expected, e.g.
AVAL
,VSSTRESN
- who_correction
WHO adjustment for weight-based indicators
A logical scalar, e.g.
TRUE
/FALSE
is expected. WHO constructed a restricted application of the LMS method for weight-based indicators. More details on these exact rules applied can be found at the document page 302 of the WHO Child Growth Standards Guidelines. If set toTRUE
the WHO correction is applied.- set_values_to_sds
Variables to be set for Z-Scores
The specified variables are set to the specified values for the new observations. For example,
set_values_to_sds(exprs(PARAMCD = "WGTHSDS", PARAM = "Weight-for-height z-score"))
defines the parameter code and parameter.The formula to calculate the Z-score is as follows:
$$\frac{((\frac{obs}{M})^L - 1)}{L * S}$$
where "obs" is the observed value for the respective anthropometric measure being calculated.
Permitted Values: List of variable-value pairs
If left as default value,
NULL
, then parameter not derived in output dataset- set_values_to_pctl
Variables to be set for Percentile
The specified variables are set to the specified values for the new observations. For example,
set_values_to_pctl(exprs(PARAMCD = "WGTHPCTL", PARAM = "Weight-for-height percentile"))
defines the parameter code and parameter.Permitted Values: List of variable-value pair
If left as default value,
NULL
, then parameter not derived in output dataset
See also
Vital Signs Functions for adding Parameters/Records
derive_params_growth_age()
Examples
library(dplyr, warn.conflicts = FALSE)
library(lubridate, warn.conflicts = FALSE)
library(rlang, warn.conflicts = FALSE)
library(admiral, warn.conflicts = FALSE)
library(pharmaversesdtm, warn.conflicts = FALSE)
# derive weight for height/length only for those under 2 years old using WHO
# weight for length reference file
advs <- pharmaversesdtm::dm_peds %>%
select(USUBJID, BRTHDTC, SEX) %>%
right_join(., pharmaversesdtm::vs_peds, by = "USUBJID") %>%
mutate(
VSDT = ymd(VSDTC),
BRTHDT = ymd(BRTHDTC)
) %>%
derive_vars_duration(
new_var = AAGECUR,
new_var_unit = AAGECURU,
start_date = BRTHDT,
end_date = VSDT,
out_unit = "days"
)
heights <- pharmaversesdtm::vs_peds %>%
filter(VSTESTCD == "HEIGHT") %>%
select(USUBJID, VSSTRESN, VSSTRESU, VSDTC) %>%
rename(
HGTTMP = VSSTRESN,
HGTTMPU = VSSTRESU
)
advs <- advs %>%
right_join(., heights, by = c("USUBJID", "VSDTC"))
advs_under2 <- advs %>%
filter(AAGECUR < 730.5)
who_under2 <- bind_rows(
(admiralpeds::who_wt_for_lgth_boys %>%
mutate(
SEX = "M",
height_unit = "cm"
)
),
(admiralpeds::who_wt_for_lgth_girls %>%
mutate(
SEX = "F",
height_unit = "cm"
)
)
) %>%
rename(
HEIGHT_LENGTH = Length,
HEIGHT_LENGTHU = height_unit
)
derive_params_growth_height(
advs_under2,
sex = SEX,
height = HGTTMP,
height_unit = HGTTMPU,
meta_criteria = who_under2,
parameter = VSTESTCD == "WEIGHT",
analysis_var = VSSTRESN,
who_correction = TRUE,
set_values_to_sds = exprs(
PARAMCD = "WGTHSDS",
PARAM = "Weight-for-height/length z-score"
),
set_values_to_pctl = exprs(
PARAMCD = "WGTHPCTL",
PARAM = "Weight-for-height/length percentile"
)
)
#> # A tibble: 162 × 37
#> USUBJID BRTHDTC SEX STUDYID DOMAIN VSSEQ VSTESTCD VSTEST VSPOS VSORRES
#> <chr> <chr> <chr> <chr> <chr> <int> <chr> <chr> <chr> <chr>
#> 1 01-701-1015 2013-01… F CDISCP… VS 1 BMI BMI NA 16.577…
#> 2 01-701-1015 2013-01… F CDISCP… VS 5 BMI BMI NA 16.615…
#> 3 01-701-1015 2013-01… F CDISCP… VS 9 BMI BMI NA 16.697…
#> 4 01-701-1015 2013-01… F CDISCP… VS 13 BMI BMI NA 16.816…
#> 5 01-701-1015 2013-01… F CDISCP… VS 17 BMI BMI NA 16.824…
#> 6 01-701-1015 2013-01… F CDISCP… VS 21 BMI BMI NA 16.915…
#> 7 01-701-1015 2013-01… F CDISCP… VS 25 BMI BMI NA 17.051…
#> 8 01-701-1015 2013-01… F CDISCP… VS 29 BMI BMI NA 17.162…
#> 9 01-701-1015 2013-01… F CDISCP… VS 33 BMI BMI NA 17.248…
#> 10 01-701-1015 2013-01… F CDISCP… VS 37 BMI BMI NA 17.433…
#> # ℹ 152 more rows
#> # ℹ 27 more variables: VSORRESU <chr>, VSSTRESC <chr>, VSSTRESN <dbl>,
#> # VSSTRESU <chr>, VSSTAT <chr>, VSLOC <chr>, VSBLFL <chr>, VISITNUM <dbl>,
#> # VISIT <chr>, VISITDY <int>, VSDTC <chr>, VSDY <int>, VSTPT <chr>,
#> # VSTPTNUM <dbl>, VSELTM <chr>, VSTPTREF <chr>, VSEVAL <chr>, EPOCH <chr>,
#> # VSDT <date>, BRTHDT <date>, AAGECUR <dbl>, AAGECURU <chr>, HGTTMP <dbl>,
#> # HGTTMPU <chr>, AVAL <dbl>, PARAMCD <chr>, PARAM <chr>