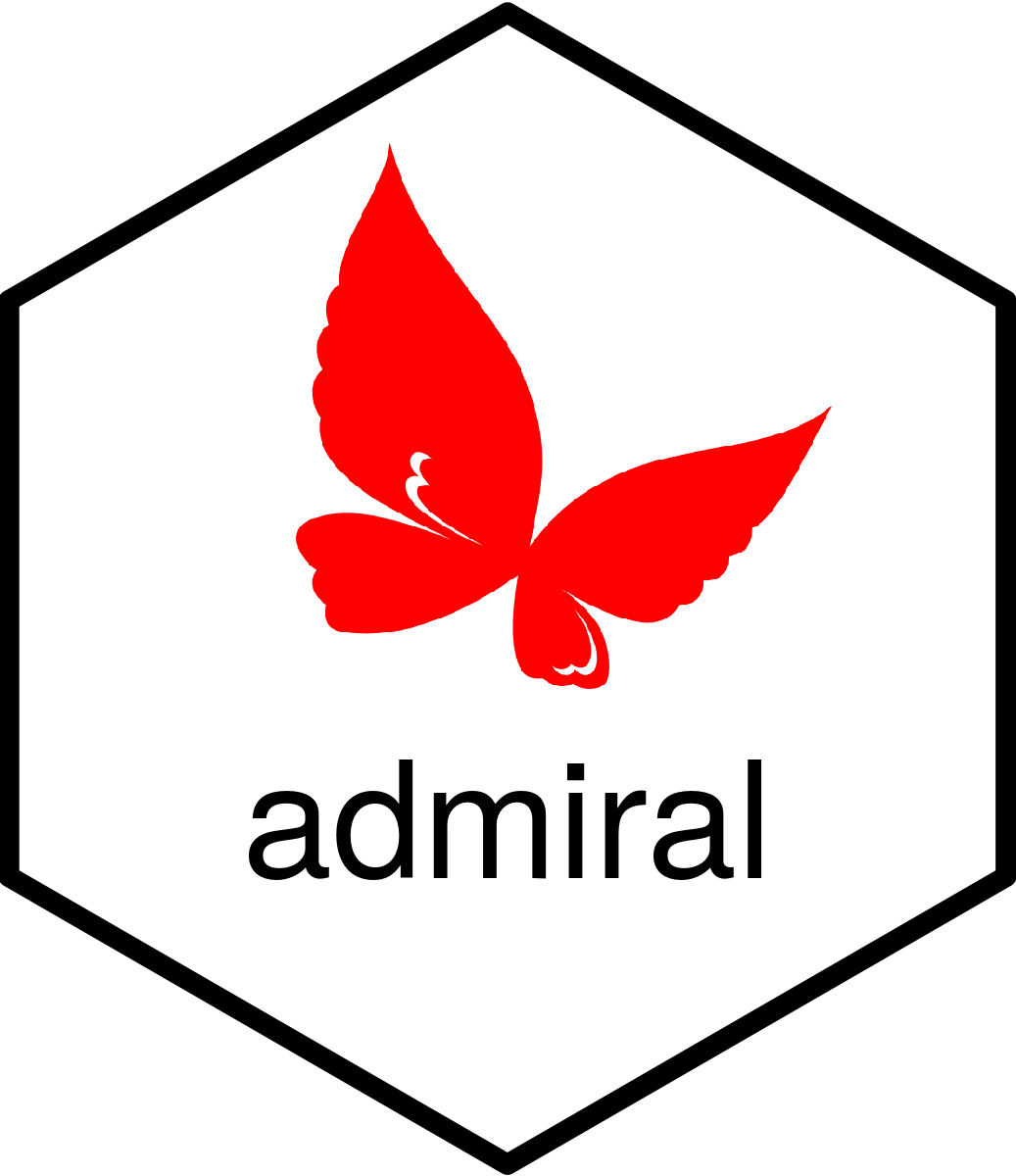
Execute a Derivation with Different Arguments for Subsets of the Input Dataset
Source:R/slice_derivation.R
slice_derivation.Rd
The input dataset is split into slices (subsets) and for each slice the derivation is called separately. Some or all arguments of the derivation may vary depending on the slice.
Arguments
- dataset
Input dataset
- derivation
Derivation
A function that performs a specific derivation is expected. A derivation adds variables or observations to a dataset. The first argument of a derivation must expect a dataset and the derivation must return a dataset. The function must provide the
dataset
argument and all arguments specified in theparams()
objects passed to thearg
argument.Please note that it is not possible to specify
{dplyr}
functions likemutate()
orsummarize()
.- args
Arguments of the derivation
A
params()
object is expected.- ...
A
derivation_slice()
object is expectedEach slice defines a subset of the input dataset and some of the parameters for the derivation. The derivation is called on the subset with the parameters specified by the
args
parameter and theargs
field of thederivation_slice()
object. If a parameter is specified for both, the value inderivation_slice()
overwrites the one inargs
.
Details
For each slice the derivation is called on the subset defined by the
filter
field of the derivation_slice()
object and with the parameters
specified by the args
parameter and the args
field of the
derivation_slice()
object. If a parameter is specified for both, the
value in derivation_slice()
overwrites the one in args
.
Observations that match with more than one slice are only considered for the first matching slice.
Observations with no match to any of the slices are included in the output dataset but the derivation is not called for them.
See also
params()
restrict_derivation()
Higher Order Functions:
call_derivation()
,
derivation_slice()
,
restrict_derivation()
Examples
library(tibble)
library(stringr)
advs <- tribble(
~USUBJID, ~VSDTC, ~VSTPT,
"1", "2020-04-16", NA_character_,
"1", "2020-04-16", "BEFORE TREATMENT"
)
# For the second slice filter is set to TRUE. Thus derive_vars_dtm is called
# with time_imputation = "last" for all observations which do not match for the
# first slice.
slice_derivation(
advs,
derivation = derive_vars_dtm,
args = params(
dtc = VSDTC,
new_vars_prefix = "A"
),
derivation_slice(
filter = str_detect(VSTPT, "PRE|BEFORE"),
args = params(time_imputation = "first")
),
derivation_slice(
filter = TRUE,
args = params(time_imputation = "last")
)
)
#> # A tibble: 2 × 5
#> USUBJID VSDTC VSTPT ADTM ATMF
#> <chr> <chr> <chr> <dttm> <chr>
#> 1 1 2020-04-16 NA 2020-04-16 23:59:59 H
#> 2 1 2020-04-16 BEFORE TREATMENT 2020-04-16 00:00:00 H